
Since we already implemented a scale_by_scalar and a length function, the implementation will be very easy: Note: every vector can be normed (or in other words: we can set every vector's length to exactly 1). This is very useful when implementing something like a move_towards function later. The interesting thing about this example is that the vectors have different lengths but they still both point into the same direction (towards the x direction). A normed vector is just a vector with the length of exactly 1. Many algorithms need normed vectors to work with directions. There are other ways to do it, but this is as easy as it gets for us, since we already implemented a sub and length function before. How it works: at first we subtract v - u and then we simply calculate the length (or length_squared).
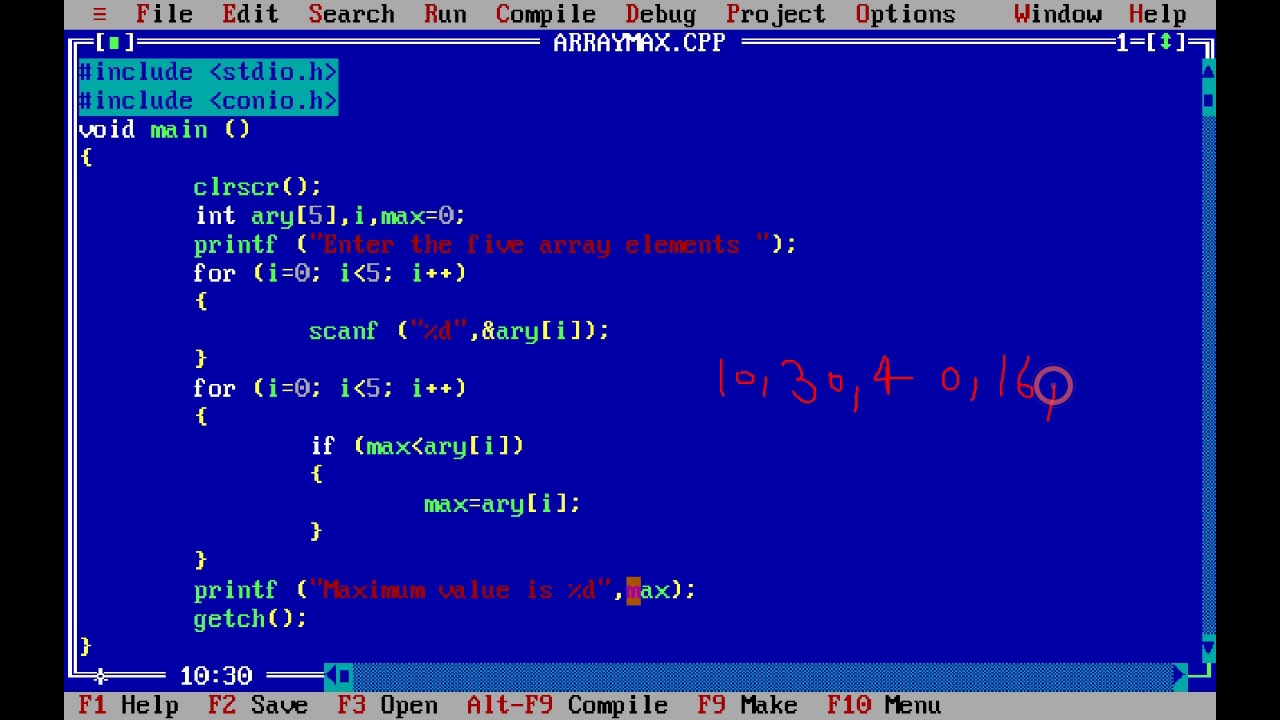
We can calculate a distance between two vectors similar to our length and length_squared functions: Print scale_by_scalar (v, 5 ) # gives Vector Distance and Dist_Squared (in other words: we multiply each component of the first vector with each component of the second vector) (in other words: we multiply each component by some value) This is done by scaling every single vector in the monster mesh. Now due to some kind of event, the monster became a boss monster which means that it should be bigger than before. Let's assume we have our typical RPG with a monster in it. If we want the real length, then we just calculate the square root of our length_squared function as seen in length. Now according to vector math, this is not the real length but the squared length. How it works: length_squared first creates a new vector with the modification a ** 2 which means a², which means a * a:Īnd then sum sums up all elements in a list, resulting in 1 + 4 = 5. As weird as it sounds, it's easier to calculate the squared length than the length: Sometimes when performance matters, we only want to know the squared length of a vector. Many algorithms need to calculate the length of a vector. It returns true if each element in the list is true.
#Python vector 2d class zip
How it works: at first we zip our values together, then we compare the tuples with each other ( a = b) which results in a list of boolean values: Often we want to find out if two vectors are equal. Print sub (u, v ) # gives Comparing Vectors The sub function is no big surprise, it's just like the add function but with a - instead of a +: Note: as mentioned before, this and all the following functions work for vector3 as well. hence for each tuple (a, b) it results in a + b: Now our list comprehension starts: we said a + b for. It creates a list where the entries of u and v are zipped together:

In the example above, the first thing that happens is zip. Let's jump right into the implementation: The reason is simple: we will use Python's list feature for our vectors:

Now instead of creating a whole vector class, we will only create a few vector functions. We will need Python's math module, hence we import it first: Print zip (u, v ) # gives Creating the ModuleĪs usual we start by creating a new module, let's name it vec.py.
#Python vector 2d class free
If you never heard of it, feel free to give our The Beauty of Python article a quick read.

We will use Python's list comprehensions and zip function.
#Python vector 2d class full
The result is a hand full of functions that work as Vector2, Vector3, Vector4 (and more) all together. The cool thing about our vector functions is that they are dimension independent. This can be done very easily with the vector functions provided in this article. A monster stands at position (x, y, z) and wants to move to a destination (x, y, z) with a certain speed. A common example for the need of vectors is monster movement. For example, in a 2D game it would be (x, y). If we want to make a game in Python, we will need vector math at some point.
